
Methods in Java Programming Language
Table of Content:
Methods in Java
A method is a set of code which is referred to by name and can be called (invoked) at any point in a program simply by utilizing the method's name. Think of a method as a subprogram that acts on data and often returns a value.
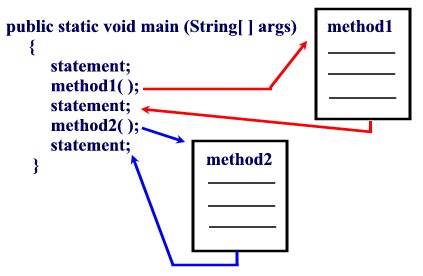
Good programmers write in a modular fashion which allows for several programmers to work independently on separate concepts which can be assembled at a later date to create the entire project. The use of methods will be our first step in the direction of modular programming.

Method definitions have four basic parts:
- The name of the method
- The type of object or base type this method returns
- A list of parameters
- The body of the method
The syntax for defining a method is:
Method definition consists of a method header
and a method body
.The same is shown in the following syntax
modifier returnValueType methodName(list of parameters) { // Method body; }
public static int methodName(int a, int b) { // body }
-
public static ? modifier
-
int ? return type
-
method name ? name of the method
-
a, b ? formal parameters
-
int a, int b ? list of parameters
method header
The method header specifies the modifiers
, return value
type, method name
, and
parameters
of the method.
-
modifier ? It defines the access type of the method and it is optional to use.
-
return type ? Method may return a value. It can be any data type.
-
name of method ? This is the method name. The method signature consists of the method name and the parameter list.
-
Parameter List ? Parameter list may two type 1. formal parameter 2. actual parameter. The list of parameters, it is the type, order, and a number of parameters of a method. These are optional, the method may contain zero parameters.
formal parameter - The variables defined in the method header are known as formal parameters or simply parameters. A parameter is like a placeholder.
actual parameter - When a method is invoked, you pass a value to the parameter. This value is referred to as an actual parameter or argument.
-
method body ? The method body defines what the method does with the statements.
method body
The method body contains a collection of statements that implement the method. The method body of the max method uses an if statement to determine which number is larger (numA or numB) and return the value of that number. In order for a value-returning method to return a result, a return statement using the keyword return is required. The method terminates when a return statement is executed.
Example of for loop
this only method part
Here is the source code of the above defined method called max(). This method takes two parameters numA and numB and returns the maximum between the two ?
public static int max(int numA, int numB) { int result; if (numA > numB){ result = numA; } else{ result = numB; } return result; }
Complete programming to calculate largest number among two number
sava program by name: MethodsExample.java
class MethodsExample{ public static void main(String args[]) { int a = 15; int b = 8; int c = max(a, b); System.out.println("Largest Value = " + c); } public static int max(int numA, int numB) { int result; if (numA > numB){ result = numA; } else{ result = numB; } return result; } }
Output:
Largest Value = 15 Press any key to continue . . .
Method Calling
In a method definition, you define what the method is to do. To execute the method, you have to call or invoke it.There are two ways in which a method is called depending on whether the method returns a value or not.
method returns a value
If a method returns a value, a call to the method is usually treated as a value. and this value will store in a variable For example,
int largestNumber = max(15, 8);
calls max(15, 8)
and assigns the result of the method to the variable largestNumber.
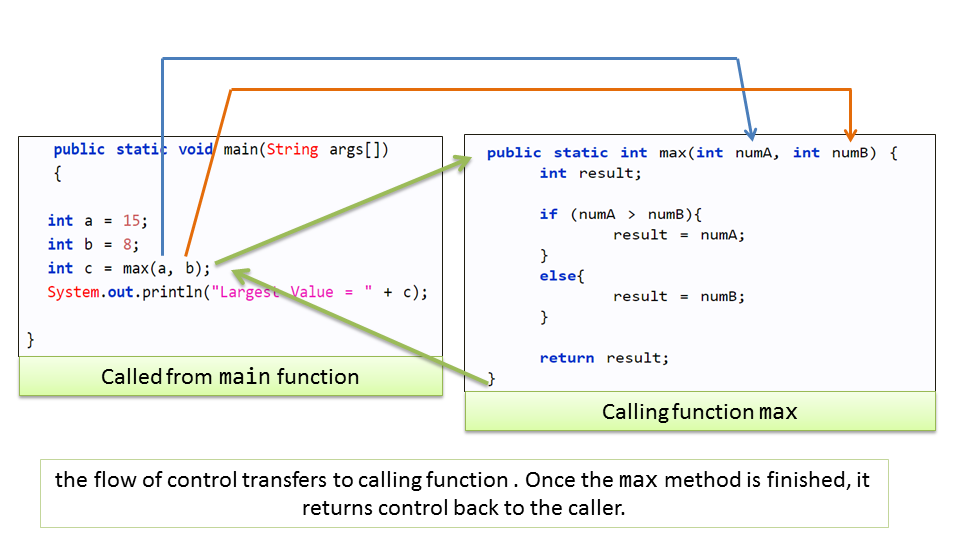
class MethodsExample{ public static void main(String args[]) { int a = 15; int b = 8; int c = max(a, b); System.out.println("The maximum of "+a+" and "+b+" is " +c); } public static int max(int numA, int numB) { int result; if (numA > numB){ result = numA; } else{ result = numB; } return result; } }Output:
The maximum of 15 and 8 is 15 Press any key to continue . . .
method returs but not in a variable
The methods returning nothing or void is considered as call to a statement. Lets consider an example ?
System.out.println(max(15, 8));
This is also a example of method calling
System.out.println("This is www.atnyla.com");
which prints the return value of the method call max(15, 8)
class MethodsExample{ public static void main(String args[]) { int a = 15; int b = 8; System.out.println("The largest number is " +max(a, b)); } public static int max(int numA, int numB) { int result; if (numA > numB){ result = numA; } else{ result = numB; } return result; } }Output:
The largest number is 15 Press any key to continue . . .
method returs nothing
class MethodsExample{ public static void main(String args[]) { int a = 15; int b = 8; max(a, b); // function calling } public static void max(int numA, int numB) { int result; if (numA > numB){ result = numA; } else{ result = numB; } System.out.println("The largest number is " +result); } }Output:
The largest number is 15 Press any key to continue . . .
Void Method
This section shows how to define and invoke a void method. The void
keyword allows us to create methods which do not return a value. Here,
in the following example we are considering a void
method printAge
.
This method is a void method, which does not return any value. Call to a void method must be a
statement i.e. printAge(78);
. It is a Java statement which ends with a semicolon as
shown in the following example. Below program that defines a method named
printAge
and invokes it to print the grade of age for a given age.
public class VoidMethodExample { public static void main(String[] args) { System.out.print("You are "); printAge(78); System.out.print("You are "); printAge(15); } public static void printAge(double age) { if (age >= 90.0) { System.out.println("lagend Old"); } else if (age >= 60.0) { System.out.println("old"); } else if (age >= 40.0) { System.out.println("adult"); } else if (age >= 20.0) { System.out.println("young"); } else { System.out.println("boy"); } } }
Output
You are old You are boy Press any key to continue . . .
Passing Parameters by Value
The power of a method is its ability to work with parameters. You can use println to print any string and max(4,7) to find the maximum of any two int values.
When you invoke a method with an argument, the value of the argument is passed to the parameter.
This is referred to as pass-by-value
.
When calling a method, you need to provide arguments, which must be given in the same order as their respective
parameters in the method signature. This is known as parameter order association
Example
public class SwappingExample { public static void main(String[] args) { int a = 3; int b = 4; System.out.println("Before swapping, a = " + a + " and b = " + b); // Invoke the swap method swapFunction(a, b); System.out.println("\n Now, Before and After swapping values will be same here :"); System.out.println("After swapping, a = " + a + " and b is " + b); } public static void swapFunction(int a, int b) { System.out.println("Before swapping(Inside), a = " + a + " b = " + b); // Swap a with b int c = a; a = b; b = c; System.out.println("After swapping(Inside), a = " + a + " b = " + b); } }
Output
Before swapping, a = 3 and b = 4 Before swapping(Inside), a = 3 b = 4 After swapping(Inside), a = 4 b = 3 Now, Before and After swapping values will be same here : After swapping, a = 3 and b is 4 Press any key to continue . . .More Examples of methods in Java Method Overloading in Java