Consume External services
Table of Content:
Consume External services
Dynamics 365 for Finance and Operations supports consuming external web services by writing a simple wrapper class on the external web service endpoint in Visual Studio and adding its reference Dynamics 365 for Finance and Operations project.
Getting ready
For this code walkthrough, we will take a free online web service for a currency converter. You can find the WSDL at http://currencyconverter.kowabunga.net/converter.asmx
How to do it.
Add a new Class Library and name it ExternalWebservices:
Add a service reference under the References node of our newly created project. Use the following service http://currencyconverter.kowabunga.net/converter.asmx .
Give a name CurrencyConverterServices in the namespace.
By clicking on the Go button, the system will fetch and show all the available services and operations in this web service, as follows:
Click on the OK button.
It will add a new node in your project service reference.
Check the app.config file. It should look as follows:
Now, go to the class1 node and add the following namespace:
Rename the class name to CurrencyConverter, and replace its main method with a new method getCurrecyRate, as shown in the following code:
Save all your code and build the project.
Sometimes, you may face unexpected errors during a build. In such cases, right-click on the solution and clean the solution. Now, try to rebuild the complete solution.
Now, our web service is ready to be consumed in Dynamics 365. Add a new Operation Project in your solution. Name it PktExternalWebServiceDAX.
In the Reference node, right-click and add a new reference. You can choose the project and it will add the dll file automatically in the reference node. See the following screenshot:
Once you click on the OK button, this must be added in the Reference node of the current project.
Now add a runnable class in your Operation Project, name it ConsumeServiceInDAX, and add a namespace of:
Add the following code in the main method:
-
Save all your code and build the solution. Set this project PktExternalWebServiceDAX as a startup project and class ConsumeServiceInDAX it as a startup Object.
-
To test the code, run the solution now. You will get your conversion rate in the following screen:
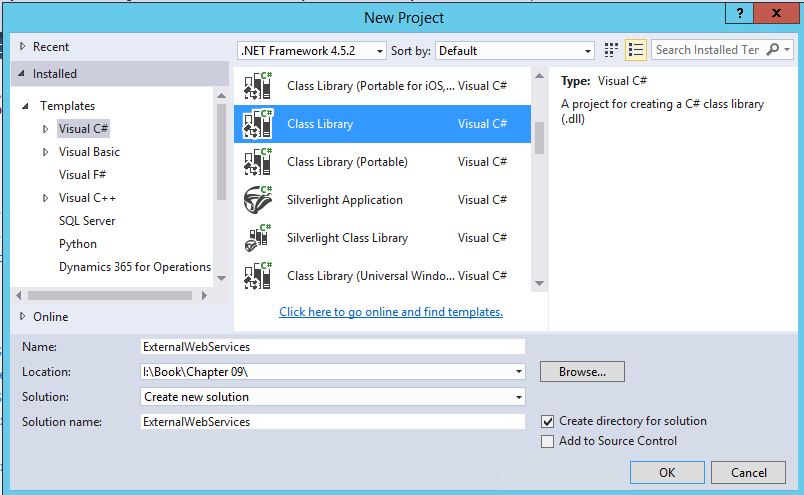
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using ExternalWebServices.CurrencyConverterService; using System.ServiceModel;
public string getCurrencyRate(String cur1, string cur2) { BasicHttpBinding httpBinding = new BasicHttpBinding(); EndpointAddress endPoint = new EndpointAddress(
@"http://currencyconverter.kowabunga.net/converter.asmx"); var soapClient = new ConverterSoapClient(httpBinding,
endPoint); var usdToinr = soapClient.GetConversionRate(
cur1.ToString(), cur2.ToString(),DateTime.Now); return usdToinr.ToString(); }
using ExternalWebServices;
ExternalWebServices.currencyConverter currencyCoverter = new ExternalWebServices.currencyConverter(); var convRate = str2Num(currencyCoverter.getCurrencyRate("USD", "INR")); info(strFmt("%1", convRate));
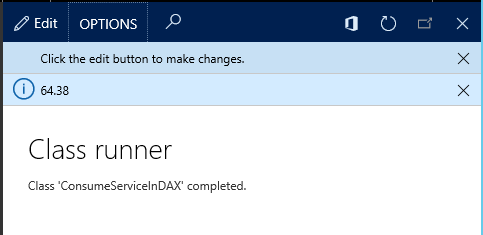
64.38 is the currency conversion rate for USD and INR.