Multilevel Inheritance in X++ Programming Language - D365 Finance and Operation
Table of Content:
Multilevel Inheritance in X++ Programming Language - D365 Finance and Operation
Multilevel inheritance in the X++ programming language, as applied in D365 Finance and Operations, offers several benefits that contribute to more organized, maintainable, and extensible code structures.
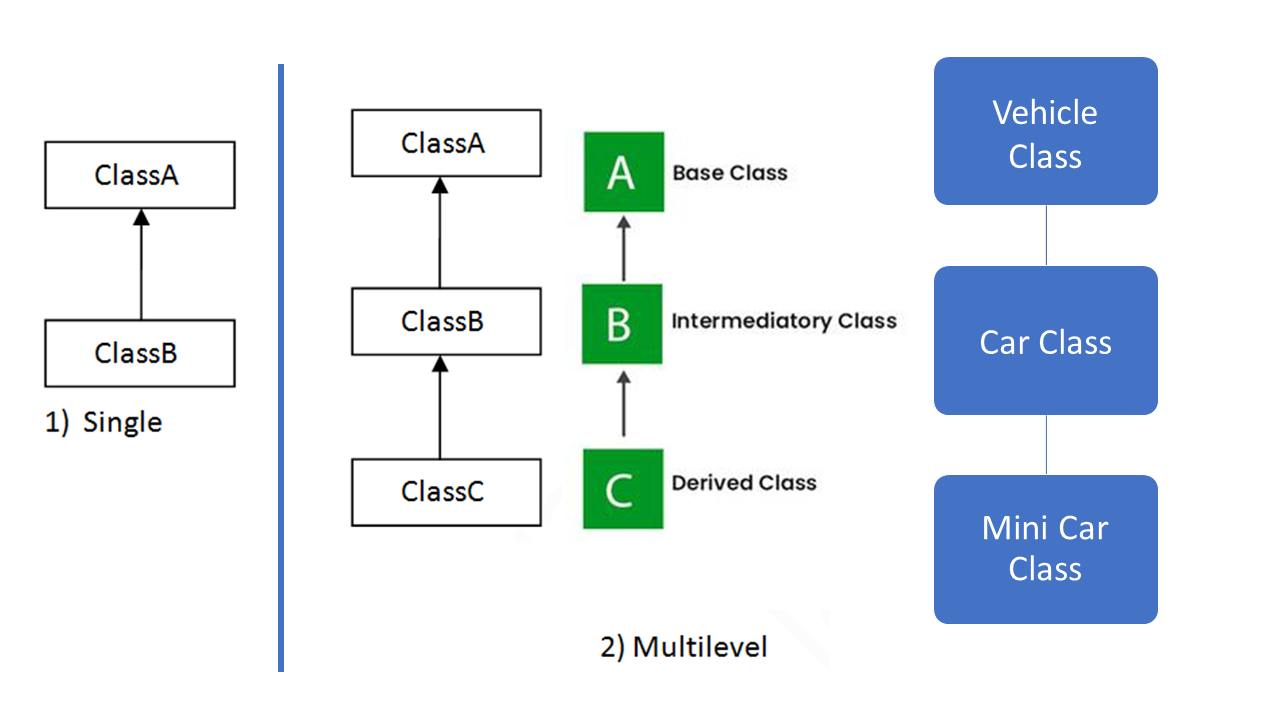
Here are some of the key benefits:
-
Code Reusability: With multilevel inheritance, you can create a hierarchy of classes where each successive class inherits attributes and behaviors from its parent class. This promotes code reuse, as common functionality can be defined in higher-level classes and inherited by subclasses. This reduces redundant code and improves development efficiency.
-
Modularity and Separation of Concerns: Multilevel inheritance allows you to break down complex systems into manageable modules. Different aspects of functionality can be encapsulated in different classes within the inheritance hierarchy. This enhances code organization, makes maintenance easier, and improves collaboration among developers.
-
Ease of Maintenance: Changes made to a parent class automatically propagate to its subclasses. This minimizes the need to update the same code in multiple places, reducing the likelihood of introducing errors and simplifying maintenance tasks.
-
Extensibility and Customization: Multilevel inheritance enables you to extend and customize existing classes to meet specific requirements. Subclasses can add new attributes and behaviors while inheriting the core functionality from their parent classes. This adaptability is particularly valuable in dynamic business environments.
-
Hierarchical Structure: Multilevel inheritance establishes a clear hierarchical relationship between classes. This makes the codebase more intuitive to understand and navigate, especially for developers who are new to the project.
-
Encapsulation and Data Hiding: By controlling access to methods and attributes through visibility modifiers (public, protected, private), multilevel inheritance supports encapsulation and data hiding. This helps prevent unintended access or modification of sensitive data and ensures the proper functioning of the classes.
-
Simplified Testing and Debugging: Code is compartmentalized into smaller, well-defined units, making it easier to isolate and test individual components. Debugging and troubleshooting become more manageable, as issues can be pinpointed to specific sections of the code.
-
Consistency and Standards: By centralizing common functionality in parent classes, you can enforce consistent behavior and coding standards across the inheritance hierarchy. This contributes to a more cohesive and maintainable codebase.
-
Scalability: Multilevel inheritance allows you to design and implement scalable solutions. As your application evolves, new subclasses can be added to extend functionality without disrupting existing code.
Parent Class
class VehicleClass { // Instance fields. real height; real width; // Constructor to initialize fields height and width. public void new(real _height, real _width) { height = _height; width = _width; } // Method public void info() { Info(strFmt("Height = %1 Width = %2", height, width)); } public void run() { Info("Vehicle class is running"); } }
First Level Child Class
class CarClass extends VehicleClass { // Additional instance field numberOfPassengers. Fields height and width are inherited. int numberOfPassengers; // Constructor is overridden to initialize. public void new(real _height, real _width, int _numberOfPassengers) { // Initialize the fields. super(_height, _width); numberOfPassengers = _numberOfPassengers; Info(strFmt("Height = %1, Width = %2, No of Passengers = %3", height, width, numberOfPassengers)); } // Method public void info() { Info(strFmt("Height = %1, Width = %2, No of Passengers = %3", height, width, numberOfPassengers)); } public void run() { super(); Info("Car class is running"); } }
Second Level Child Class
class MiniCarClass extends CarClass { // Additional instance field nameOfCar. Fields height and width are inherited. str nameOfCar; // Constructor is overridden to initialize. public void new(real _height, real _width, int _numberOfPassengers, str _nameOfCar) { // Initialize the fields. super(_height, _width, _numberOfPassengers); nameOfCar= _nameOfCar; Info(strFmt("Height = %1, Width = %2, No of Passengers = %3, Name of Car = %4", height, width, numberOfPassengers, nameOfCar)); } // Method which will display the name of the car public void displayNameOfCar() { Info(strFmt("Name of the Car is = %1", nameOfCar)); } public void run() { super(); Info("MiniCar class is running"); } }
Create object and run the code
internal final class TestClass { /// /// Class entry point. The system will call this method when a designated menu /// is selected or when execution starts and this class is set as the startup class. /// /// The specified arguments. public static void main(Args _args) { MiniCarClass objMiniCar = new MiniCarClass (10.0, 12.0, 2, "Car no 1"); objMiniCar.displayNameOfCar(); objMiniCar .info(); objMiniCar .run(); } }
In summary, multilevel inheritance in the X++ programming language, when used in D365 Finance and Operations, offers a powerful mechanism for creating modular, flexible, and maintainable codebases. It promotes best practices in software design and development, enhancing the quality and longevity of your applications.