
Arithmetic operators in Python
Table of Content:
Arithmetic operators
Arithmetic operators are used to perform arithmetic operations between two operands. It includes +(addition), - (subtraction), *(multiplication), /(divide), %(reminder), //(floor division), and exponent (**).
Consider the following table for a detailed explanation of arithmetic operators.
Operator | Description |
---|---|
+ (Addition) | It is used to add two operands. For example, if a = 20, b = 10 => a+b = 30 |
- (Subtraction) | It is used to subtract the second operand from the first operand. If the first operand is less than the second operand, the value result negative. For example, if a = 20, b = 10 => a - b = 10 |
/ (divide) | It returns the quotient after dividing the first operand by the second operand. For example, if a = 20, b = 10 => a/b = 2 |
* (Multiplication) | It is used to multiply one operand with the other. For example, if a = 20, b = 10 => a * b = 200 |
% (reminder) | It returns the reminder after dividing the first operand by the second operand. For example, if a = 20, b = 10 => a%b = 0 |
** (Exponent) | It is an exponent operator represented as it calculates the first operand power to second operand. |
// (Floor division) | It gives the floor value of the quotient produced by dividing the two operands. |
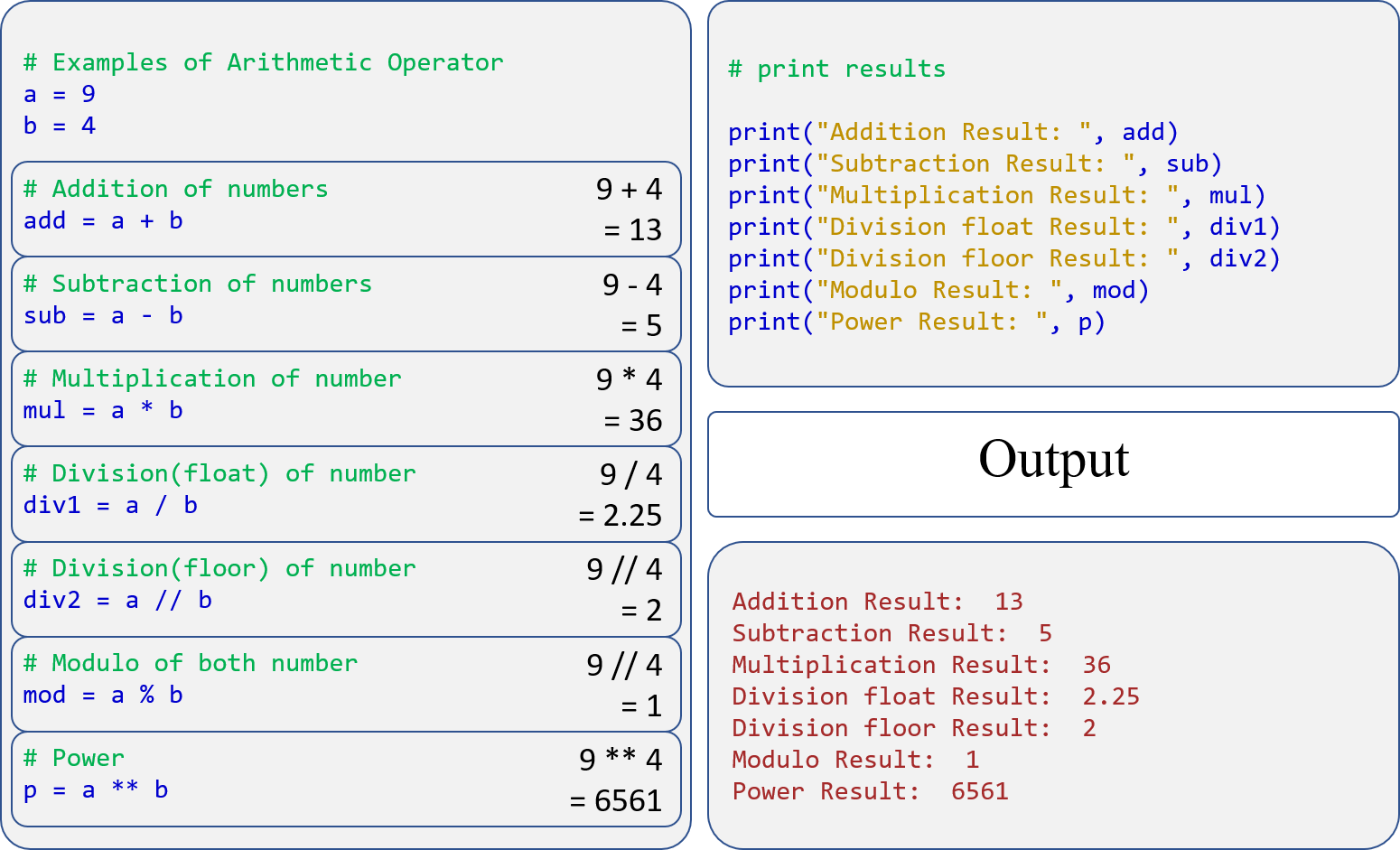
Code
# Examples of Arithmetic Operator a = 9 b = 4 # Addition of numbers add = a + b # Subtraction of numbers sub = a - b # Multiplication of number mul = a * b # Division(float) of number div1 = a / b # Division(floor) of number div2 = a // b # Modulo of both number mod = a % b # Power p = a ** b # print results print("Addition Result: ", add) print("Subtraction Result: ", sub) print("Multiplication Result: ", mul) print("Division float Result: ", div1) print("Division floor Result: ", div2) print("Modulo Result: ", mod) print("Power Result: ", p)
Output
Addition Result: 13 Subtraction Result: 5 Multiplication Result: 36 Division float Result: 2.25 Division floor Result: 2 Modulo Result: 1 Power Result: 6561