
Char Data Type in Java Programming Language
Table of Content:
char Data Type in Java
In Java, the data type used to store characters is char
. A character data type represents a single character.
A character is an identifier which is enclosed within single quotes. In java to represent character data, we use
a data type called char
.This data type takes two byte (16-bit) since it follows Unicode character set.The 16-bit Unicode character
set underlies both the Java source program and char data type. So, not only are Java programs written in Unicode characters,
but Java programs can manipulate Unicode data.
- char data type is a single 16-bit Unicode character
- Minimum value is '\u0000' (or 0)
- Maximum value is '\uffff' (or 65,535 inclusive)
- Char data type is used to store any character
- Example:
char letter = 'A'; char numChar = '5';
The first statement assigns character A to the char variable letter . The second statement assigns digit character 5 to the char variable numChar
Data Type | Size(Byte) | Range |
---|---|---|
Char | 2 | 232767 to -32768 |
char Variable Declaration and Variable Initialization:
Variable Declaration : To declare a variable , you must specify the data type & give the variable a unique name.
char flag ;
Variable Initialization : To initialize a variable you must assign it a valid value.
flag = 'a' ;
char Variable Declaration and Initialization in two step:
char flag ; flag = 'a' ;
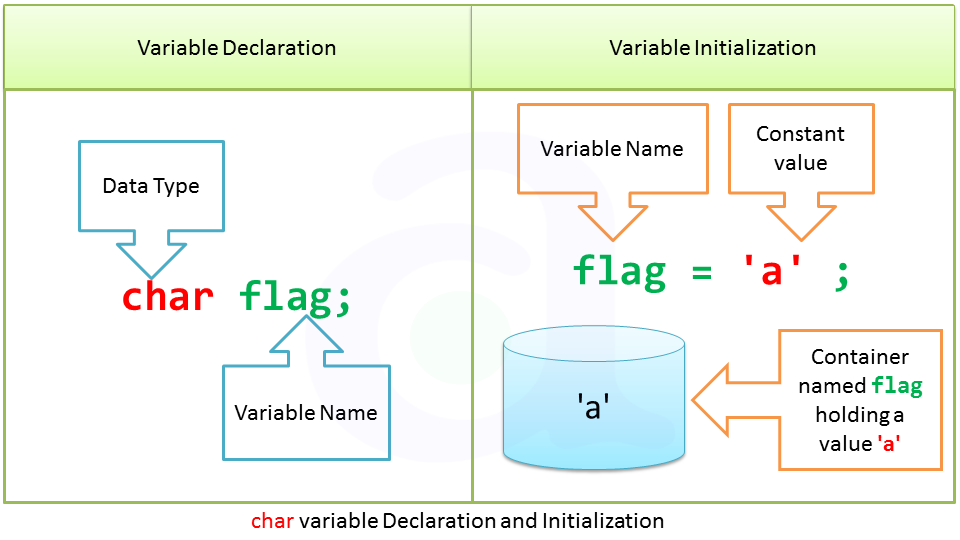
Example :
Save Source File Name as : CharacterExample1.javaTo compile : javac CharacterExample1.java
To Run : java CharacterExample1
// A Java program to demonstrate char data type class CharacterExample1 { public static void main(String args[]) { char flag; flag = 'a' ; System.out.println(flag); } }Output
a Press any key to continue . . .

char Variable Declaration and Initialization in single step:
char flag = 'a' ;
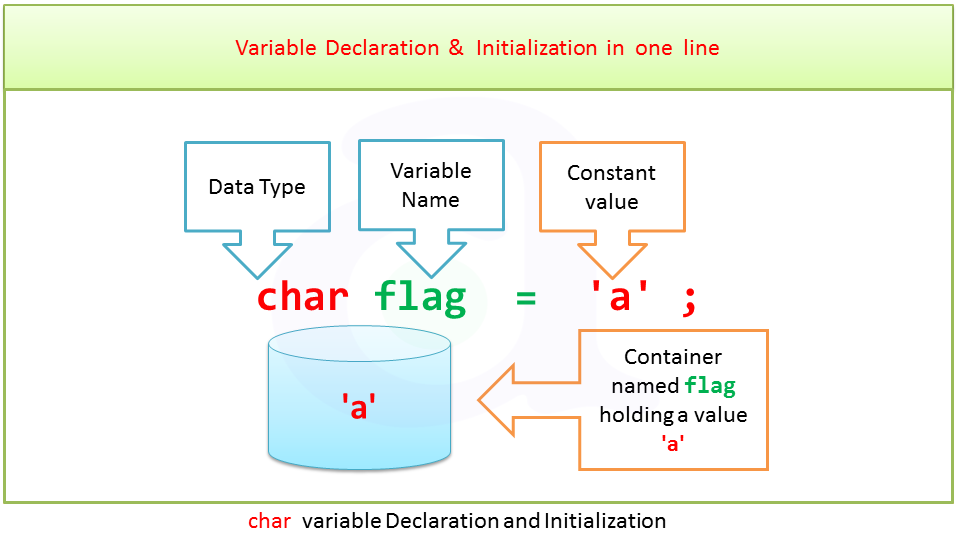
Example :
// A Java program to demonstrate char data type class CharacterExample1 { public static void main(String args[]) { char flag = 'a' ; System.out.println(flag); } }Output
a Press any key to continue . . .
A program that demonstrates char variables:
Save Source File Name as : CharacterExample2.javaTo compile : javac CharacterExample2.java
To Run : java CharacterExample2
// Demonstrate char data type. class CharDemo { public static void main(String args[]) { char character1, character2; character1 = 82; // code for X character2 = 'S'; System.out.print("character1 and character2: "); System.out.println(character1 + " " + character2); } }Output
character1 and character2: R S Press any key to continue . . .
Explanation
Notice that character1
is assigned the value 82, which is the ASCII (and Unicode) value that
corresponds to the letter R. As mentioned, the ASCII character set occupies the first 127
values in the Unicode character set.
char is designed to hold Unicode characters, it can also be thought of as an integer type on which you can perform arithmetic operations.
// char variables behave like integers. class CharDemo { public static void main(String args[]) { char character1 ; character1 = 'R'; System.out.println("ch1 contains " + character1 ); character1++; // increment character1 System.out.println("ch1 is now " + character1 ); } }Output
ch1 contains R ch1 is now S Press any key to continue . . .