
Hello World Program in Vue JS
Table of Content:
The Formal Start
-
In this topic, you will understand the difference between a dynamic web-page and a static web-page.
-
For this, you will build a minimalistic HTML and JS code to display Hello World! on the browser, without Vue.
-
After that, Vue code will be added (using CDN) and tested using browser developer console to test the dynamic nature of the web-page.
HTML and JS
Vue JS works by binding JS with HTML and optionally with CSS. Consider the sample HTML and JS code without Vue part in it, as given below. After that, to understand the difference, Vue will be added.
Loading...
// main.js var message = "Hello World!"; window.onload = function() { document.querySelector('h1').innerHTML = message; };
Output without Vue
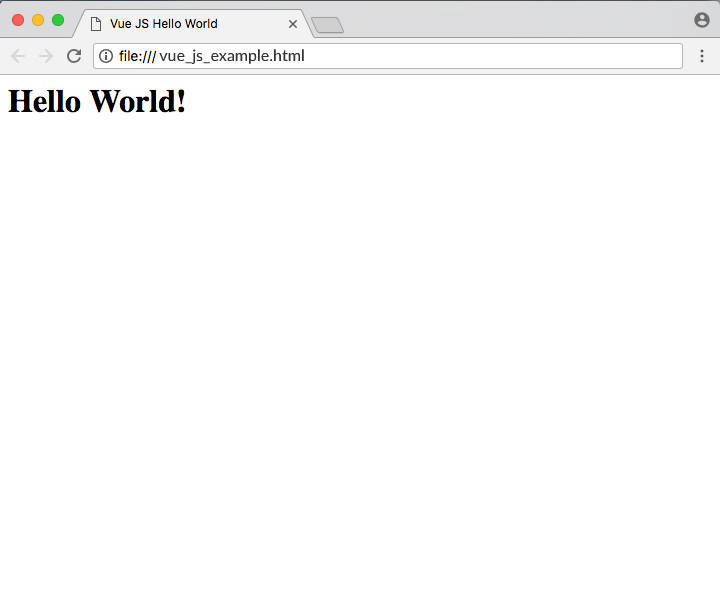
Adding the magic
Now add the magic potion from Vue JS and check how the JS variables can be used in HTML.
The magic requires to include the Vue JS file from it's CDN.
{{ message }}
// main.js var app = null; window.onload = function() { app = new Vue({ el: '#vueMain', data: { message: 'Hello World!' } }); };
Note: Do not use the body tag's ID to refer to root element.
Testing the Power of magic
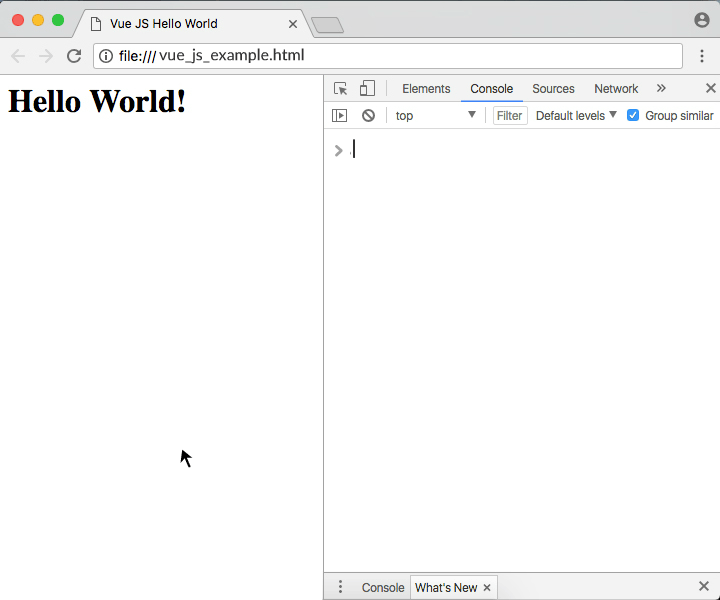
Revealing the Secret of magic
-
Vue instance is the root of the application.
-
It is created by specifying various options to store data and perform actions.
-
Vue instance is plugged into the
el
attribute forming a relationship between the instance and the portion of HTML element. -
The 'data' attribute allows you to access the variables for that instance.
Note: In the first example without Vue, execute
message = "Hi All!"
in the developer console. The HTML will not get updated.
A Visual Explanation
The given image explains how JS data is being used in HTML files.
