A complete overview of Runtime Compilation in ASP.NET Core
In this blog, we will discuss on Runtime Compilation in Asp.Net Core. Runtime Compilation also called a Razor File Compilation. If you have configured Razor File Compilation in your project, you would have below prerequisites.
Prerequisite for Razor File Compilation:
- Visual Studio 2019
- .NET SDK 3.1 or later
Razor File Compilation: -> Razor files with a .cshtml extension you can compile build and publish time by using Razor SDK. Runtime Compilation may be optionally enabling by configuring your project.
Razor Compilation: -> When your project build and publish time compilation of Razor Files is enabled by default Razor SDK. If your Razor Files are updated or edited it would be allowed to Runtime Compilation.
Enable Runtime Compilation at Project Creation: -> You can enable Runtime Compilation at Project Creation in two ways:
- In Visual Studio
- Create a .NET Core Web Application.
- Select either the Web Application or the Web Application (Model - View - Controller) project template.
- Select the Enable Razor Runtime Compilation checkbox.
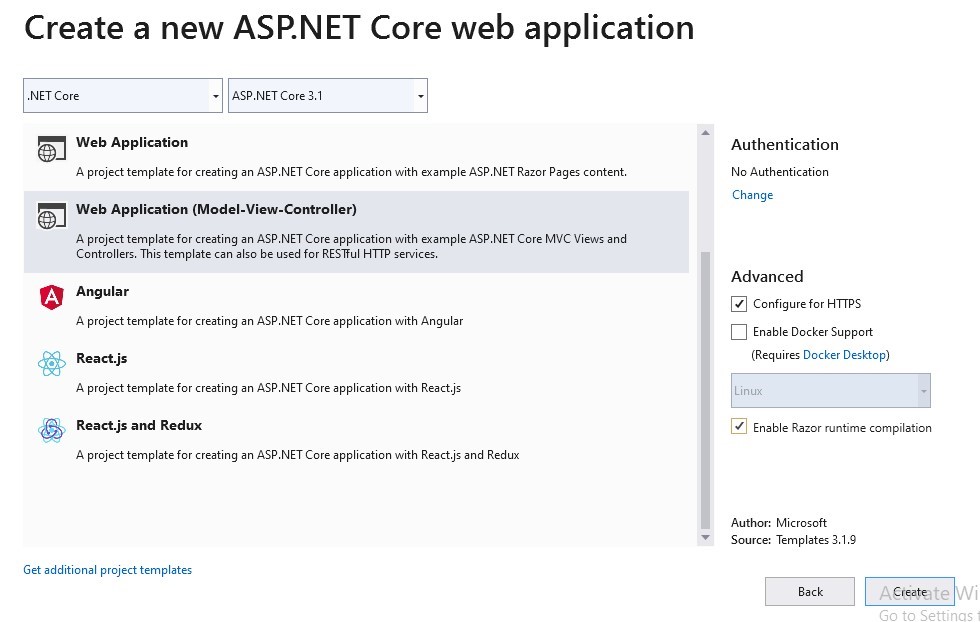
2. In .NET Core CLI
- If you create a new Razor project with runtime compilation enabled, you can use these command -rrc or --razor-runtime-compilation
- dotnet new webapp --razor-runtime-compilation
Enable Runtime Compilation in an Existing Project.
All environment to configure in enable Runtime Compilation in an existing project:
- Install Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation
- Right click on project -> Manage NuGet packages -> Browse -> Search the AspNetCore.Mvc.Razor.RuntimeCompilation -> Install
- Update the project’s Startup.ConfigureServices method to include a call to AddRazorRuntimeCompilation for example:

Conditionally Enable Runtime Compilation In An Existing Project.
The Runtime Compilation can be enabled conditionally such that it’s only available for local development.
Conditionally enabling in this manually ensures that the published output-
- Uses compiled views
- Doesn’t enable file watchers in production.
You can enable Runtime Compilation only in the Development environment.
- Install Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation
- Right click on project -> Manage NuGet packages -> Browse -> Search the Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation -> Install
- Modify the launch profile environmentVariables section in launchSettings.json:
- Verify ASPNETCORE_ENVIRONMENT is set to “Development”
- Set ASPNETCORE_HOSTINGSTARTUOASSEMBLIES to “Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation”
In the following example, Runtime compilation is enabled in the Development environment for the IIS Express and RazorPagesApp launch profile:
No need to make code changes in the project’s Startup class. While Runtime ASP.NET Core searches for an assembly-level HostingStartup Attribute in Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation. The HostingAttribute specifies to Startup code to execute the app.
{ "iisSettings": { "windowsAuthentication": false, "anonymousAuthentication": true, "iisExpress": { "applicationUrl": "http://localhost:62303", "sslPort": 44370 } }, "profiles": { "IIS Express": { "commandName": "IISExpress", "launchBrowser": true, "environmentVariables": { "ASPNETCORE_ENVIRONMENT": "Development", "ASPNETCORE_HOSTINGSTARTUPASSEMBLIES": "Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation" } }, "RuntimeCompile": { "commandName": "Project", "launchBrowser": true, "applicationUrl": "https://localhost:5001;http://localhost:5000", "environmentVariables": { "ASPNETCORE_ENVIRONMENT": "Development", "ASPNETCORE_HOSTINGSTARTUPASSEMBLIES": "Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation" } } } }
Your Startup code enables Runtime Compilation.
Enable Runtime Compilation for a Razor Class Library :-> The Razor Pages references the Razor Class Library (RCL) named which consider a scenario in MyClassLib. The RCL contains a _Layout.cshtml file that when you create a Web Application that includes the MVC and Razor projects consume. The _Layout.cshtml in RCL so you want to enable Runtime Compilation so that you make following changes in the Razor Pages project:
- Conditionally enable Runtime Compilation in an existing project to enable Runtime Compilation with the instructions.
- ConfigureServices options configure the Runtime Compilation.
Example: Startup.cs
public void ConfigureServices(IServiceCollection services) { services.AddControllersWithViews(); services.AddRazorPages().AddRazorRuntimeCompilation(); services.AddRazorPages(); services.Configure (options => { var libraryPath = Path.GetFullPath( Path.Combine(HostEnvironment.ContentRootPath, "..", "MyClassLib")); options.FileProviders.Add(new PhysicalFileProvider(libraryPath)); }); }
The PhysicalFileProvider API is located and used from directories and files at the absolute path to MyClassLib RCL gets Constructed. The PhysicalFileProvider instance is added to file providers collection, which allows access to the RCL’s .cshtml files. To when you described in the Startup.ConfigureServices method.
Razor Class Library: Razor components, View components, Razor Pages, Views, Page Models, Controllers and Data Models all you can built with Razor Class Library(RCL). The RCL can be Packaged and reused. The Application can include the RCL and override the views and pages. When a view, Partial view, Razor Pages are included for the app, RCL, the Razor markup (.cshtml file ) in the app takes precedence.
Create A Class Library Containing Razor UI: You can create this in two ways.
- Visual Studio 2019
- Create a New Project
- Select the Razor Class Library -> Next.
- Name the Library (For Example “RazorClassLib”) -> Create. Avoid a file name collision with a new library. Your library name does not end with the (.Views)
- Select Support pages and views. If you need to support views, By Default, only Razor Pages are supported -> Create
- .Net Core CLI
- dotnet new razorclasslib -o RazorUIClassLib
Add Razor Files to the RCL: The RCL would be placed in the Areas folder. You can find the files in ~/Pages rather than ~/Areas/Pages.
Compile the Views Only for Debug: This is an excellent feature supported in the Visual Studio and .NET. You can just add “#if(DEBUG)” in the code and add the Runtime Compilation only if you can debug to the code. Because of this service, we can focus more on productivity in the production environment with smooth debugging.
Example:
public void ConfigureServices(IServiceCollection services) { var views = services.AddControllersWithViews(); #if (Debug) views.AddRazorRuntimeCompilation #endif }
You can create the app to configure with enable Only in Razor Runtime Compilation to Debug environment using Debug environment variable, like this
Example:
public IWebHostEnvironment webHost { get; set; } public IConfiguration Configuration { get; } // This method gets called by the runtime. this method to add services to the container of ConfigureServices Method. public void ConfigureServices(IServiceCollection services) { var views = services.AddControllersWithViews(); #if (Debug) if(webHost.IsDevelopment()){ views.AddRazorRuntimeCompilation(); } #endif }
In Startup file, you have Startup Constructor here, where the IWebHostEnvironment will be injected and sets the webhostenvironment property.
Example:
public Startup(IConfiguration configuration, IWebHostEnvironment environment) { Configuration = configuration; webHost = environment; }
Conclusion
In this blog, we have seen the detailed overview of Runtime compilation support in Asp.Net Core. We have learned the utilization of Razor file with RCL. Hope this would help you.