
Two-dimension Array in Data Structure
Table of Content:
Note: Before going to the array in data structure please read the basic array concept from our c programming tutorial here is the link:
A two-dimension m x n array, A is it collection of m.n data elements such that each elements is specified by pair of integers (such as J,K) called subscripts, with the property that,
1<= J <= m , 1<= K <= n
There is a standard way of drawing a two-dimensional m x n array A where, the elements of A form a rectangular array with m rows and n columns and where, the elements A[J.K] appears in row J and column K,
Suppose, A is a two-dimensional m x n array. The first dimension of A contains the index set 1, ... m with lower bound 1 and upper bound m, and the second dimension of A contains the index set 1, 2, ... n with lower bound 1 and upper bound n. The length of a dimension is the n0 of integers in its index set. The pair of lengths m x n is called size of the array.
Programming language allows one to define multi-dimensional array in which lower bounds are not 1. However, the index set for each dimension is still consists of consecutive integers from the lower bound to the upper bound of the dimension. The length of a given dimension can be obtained from the formula,
Length = UB - LB + 1
For two-dimensional array we can find the length of the array by the following procedure,
- First we will find the length of one-dimensional row array by the formula,
- Do this again one-dimensional column array.
- Length of array (number of elements in two-dimensional array)
L1 =UB1 - LB1 + 1
L2 =UB2 - LB2 + 1
L = L1x L2
Example
Given array int A(2 : 5, - 3 : 1)
- The lengeh of row one-dimensional array (5 - 2 +1 = 4)
- The length of column one-dimensional array (1 - (-3) +1=5)
- Length of given array = 4 x 5 = 20
So, there are 20 elements on the given array.
Representation of two-dimensional array in memory
Let, A be a two-dimensional array m x n array. Although A is pictured as a rectangular array of elements with m rows and n columns, the array will be represented in memory by a block m.n sequential memory location. Specifically, the propgramming language will store the array A either,
- column by column is called column major order or
- Row by row, in row major order
Given Fig. 3. shows these two ways when A is a two-dimensional 3 x 4 array. We emphasize that the particular representation used depends upon the programming language, not the user.
Recall that, for a linear array, the computer does not keep track of the address LOC(LA[K]) of every element LA[K]
of LA, but does keep track of Base (LA) the address of the first element of LA. The computer uses the formula.
LOC[LA[K]] = Base(LA) +W(K-1)
For 3x4 array Column Major Order (CMO)

For 3x4 array Row Major Order (RMO)
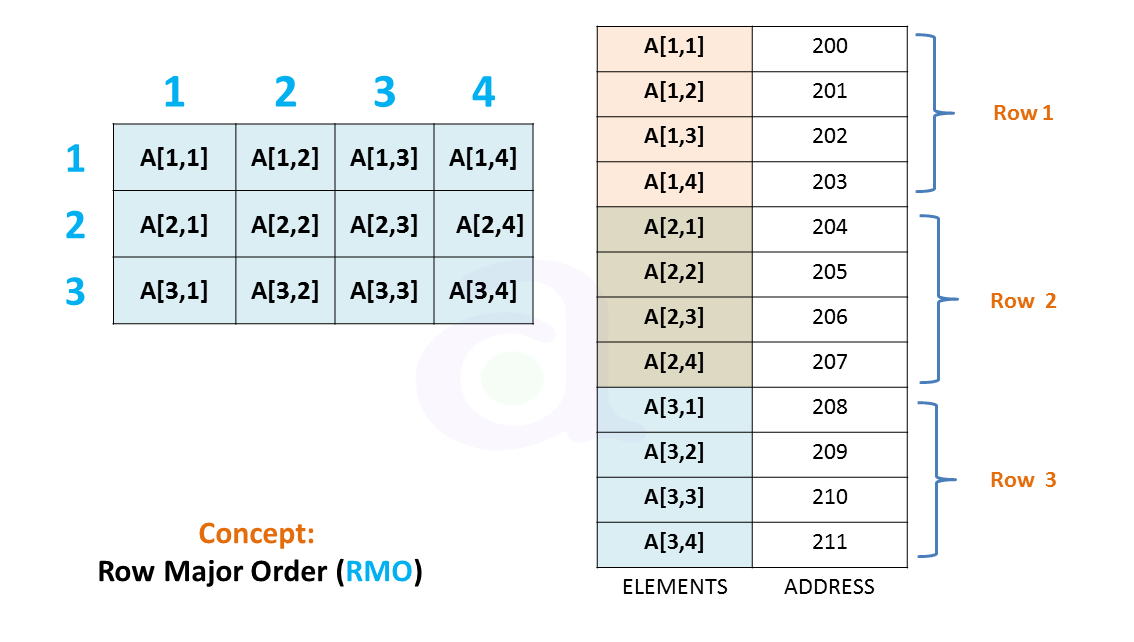
To find the address of LA[K] in time independent of K. Here, w is the number of words per memory cell for the array LA, and 1 is the lower bound of the index set of LA.
A similar situation also holds for any two-dimensional m x n array A. That is, the computer keeps track of base [A], the address of the first element A[1, 1] of A - and computes the address LOC (A[J, KJ) of A[J, K] using the formula,
(Column major order)
LOC(A[J,K])=Base(A)+w(A(K-1)+(J-1)]
(Row major order)
LOC (A[J, K]) = Base (A) + w [N(J - 1) + (K - 1)]
Again, w denotes the number of words per memorY location for the array A . Note that the formula is linear in J and K and that one can find the address LOC (A[J, K]) in time independent of J and K.
Example
Consider the 25 x 4 matrix array SCORE. Suppose, base (SCORE) = 200 and there are w = 4 words Per memory cell. Furthermore, suppose the programming_ language stores two-dimensional array using row
order. The address of SCORE [12, 3] follows,
LOC (SCORE [12, 3]) = 200 + 4 [4(12 -1) + (3-1)] = 200 + 4 [46] = 384