
Class Introduction X++ Programming Language
Table of Content:
Class, data, method and Object
A class is a software construct that defines the data and methods of the instances that are later constructed from that class. The class is an abstraction of an object in the problem domain. The instances that are constructed from the class are known as instances or objects. This topic uses the term instance. The data represents the state
of the object, whereas the methods represent the behavior
of the object. Variables contain the data for the class. Every instance that is constructed from the class declaration has its own copy of the variables. These variables are known as instance variables. Methods define the behavior of a class. They are the sequences of statements that operate on the data (instance variables). By default, methods are declared to operate on the instance variables of the class. These methods are known as instance methods or object methods.
You can add a class to your project in Solution Explorer. Additionally, you can select the type of class that you want to create during this process.
How to Add a Class In Visual Studio Project
- In Visual Studio, while running as an administrator, go to Solution Explorer.
- Right-click your project.
- Select Add > New Item.
- Under Dynamics 365 Items, select Code.
- Select either Class or Runnable Class (Job), depending on the purpose of the class.
- Enter a name for the class.
- Select Add to add the class to your project.
To tun a simple hello word program through class, we need this below code.
Serial no | important |
1 | Class Declaration |
2 | Main Method |
class Declaration
class Class1 { }
Main Method
Main method - Creates an instance of an object and calls the required member methods. It is a class method that is run directly from a menu option. To transfer data to the method, use the _args
parameter. The following is an example of the syntax that is used to define a main method:
public static void main(Args _args) { info("Hello World!"); }
Full Code which is executable
class Class1 { public static void main(Args _args) { info("Hello World!"); } }
Instance method
Instance method - Also referred to as an object method, it is embedded in each object that is created from the class that contains the instance method. Before you can use the method, you must instantiate that object. The following is an example of the syntax that is used to call an instance method:
ClassName objectReference = new ClassName(); objectReference.methodname();
Static method
Static method - Also referred to as a class method, it uses the static
keyword and belongs to a class. With a static method, unlike with an instance method, you don't need to instantiate an object before you use the method. Static methods are commonly used to work with data that is stored in tables. The following is an example of the syntax that is used to call a static method:
ClassName::methodName();
Runnable Class
A runnable class for finance and operations apps, known previously as a job, is similar to a standard class, except that it contains a main
method. You can manually add methods to your class, including a main method. Methods will be described in further detail in the next unit. The following screenshot shows the code editor window when you create a runnable class named RunnableClass1
.
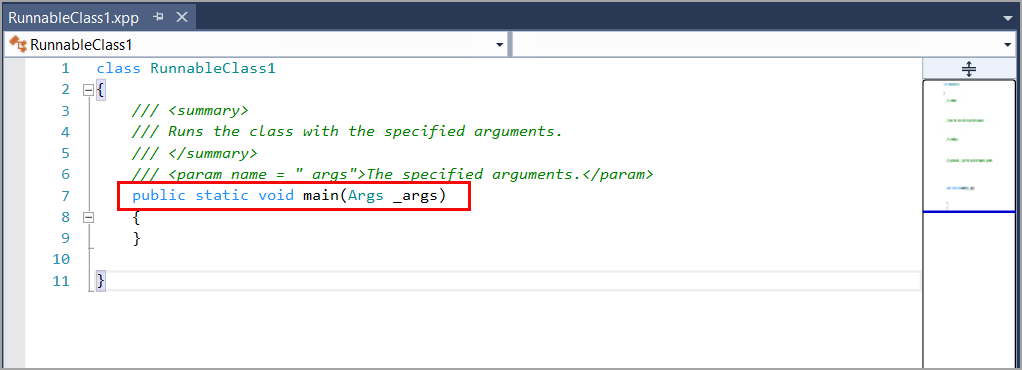
Important Points about Class
- Classes contain data and methods that represent the state and the behavior of an object.
- Some elements in the Application Object Tree (AOT), such as tables and forms, contain classes out of the box. Many types of methods and statements can be called to dictate the behavior of objects.
- Classes can be added to a project to declare new elements, variables, or methods. You can also use classes to manipulate data, such as inserting or deleting data from a table. You can comment on code in new classes to help define the purpose of the block of code. This is helpful when you are working as part of a team to increase collaboration and communication.
- To fit your business needs, you will write code in a class to adjust the functionality of finance and operations apps.